Switch statement
Introduction
The switch statement is similar to the series of if-else statements. The switch statement performs in various cases i.e. it has various cases to which it matches the condition and appropriately executes a particular case block. It first evaluates an expression and then compares it with the values of each case. If a case matches then the same case is executed.
Syntax:
The switch
statement compares an expression
with the value in each case.
If the expression equals a value in a case, e.g., value1
, PHP executes the code block in the matching case until it encounters the first break
statement.
If there’s no match and the default
is available, PHP executes all statements following the default
keyword.
In case the default
is not specified, and there’s no match, the control is passed to the statement that follows the switch
statement.
Combining cases
Since PHP executes the switch
statement from the matching case label till it encounters the break
statement, you can combine several cases in one.
The following example uses the switch statement and combines the cases of 'editor'
and 'author'
:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 | <?php $message = ''; $role = 'author'; switch ($role) { case 'admin': $message = 'Welcome, admin!'; break; case 'editor': case 'author': $message = 'Welcome! Do you want to create a new article?'; break; case 'subscriber': $message = 'Welcome! Check out some new articles.'; break; default: $message = 'You are not authorized to access this page'; } echo $message;//Welcome! Do you want to create a new article? |
For statement
Introduction
The for
statement allows you to execute a code block repeatedly.
Syntax:
- The
start
is evaluated once when the loop starts. - The
condition
is evaluated once in each iteration. If thecondition
istrue
, thestatement
in the body is executed. Otherwise, the loop ends. - The
increment
expression is evaluated once after each iteration.
When you leave all three parts empty, you should use a break
statement to exit the loop at some point. Otherwise, you’ll have an infinite loop:
1 2 3 4 5 6 7 8 9 | <?php $total = 0; for ($i = 1; $i <= 10; $i++) { $total += $i; } echo $total; //55 |
- First, initialize the
$total
to zero. - Second, start the loop by setting the variable
$i
to 1. This initialization will be evaluated once when the loop starts. - Third, the loop continues as long as
$i
is less than or equal to10
. The expression$i <= 10
is evaluated once after every iteration. - Fourth, the expression
$i++
is evaluated after each iteration. - Finally, the loop runs exactly
10
iterations and stops once$i
becomes11
.
Alternative syntax
Syntax:
statement;
endfor;
1 2 3 4 5 6 7 8 9 | <?php $total = 0; for ($i = 1; $i <= 10; $i++): $total += $i; endfor; echo $total; //55 |
While statement
Introduction
The while
statement executes a code block as long as an expression
is true
.
Syntax:
- First, PHP evaluates the
expression
. If the result istrue
, PHP executes thestatement
. - Then, PHP re-evaluates the
expression
again. If it’s stilltrue
, PHP executes the statement again. However, if theexpression
isfalse
, the loop ends.
Ex:
1 2 3 4 5 6 7 8 9 10 11 | <?php $total = 0; $number = 1; while ($number <= 10) { $total += $number; $number++; } echo $total; //55 |
Alternative syntax
1 2 3 4 5 6 7 8 9 10 11 | <?php $total = 0; $number = 1; while ($number <= 10) : $total += $number; $number++; endwhile; echo $total; //55 |
do … while statement
Introduction
The PHP do...while
statement allows you to execute a code block repeatedly based on a Boolean expression.
while
statement, PHP evaluates the expression
at the end of each iteration. It means that the loop always executes at least once, even the expression
is false
before the loop enters.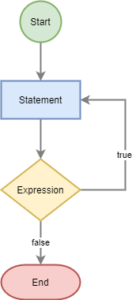
In the following example, the code block inside the do...while
loop executes ten times:
1 2 3 4 5 6 7 8 | <?php $i = 10; do { echo $i . '<br>'; $i--; } while ($i > 0); |
do…while vs. while
The differences between the do...while
and while
statements are:
- PHP executes the statement in
do...while
at least once, whereas it won’t execute thestatement
in thewhile
statement if theexpression
isfalse
. - PHP evaluates the
expression
in thedo...while
statement at the end of each iteration. Conversely, PHP evaluates theexpression
in thewhile
statement at the beginning of each iteration.
Foreach statement
Introduction
The foreach
statement iterates over all elements in an array, one at a time. It starts with the first element and ends with the last one. Therefore, you don’t need to know the number of elements in an array upfront.
With indexed arrays
Syntax:
<?php
foreach ($array_name as $element) {
// process element here
}
When PHP encounters a foreach
statement, it assigns the first element of the array to the variable following the as
keyword ($element
).
In each iteration, PHP assigns the next array element to the $element
variable. If PHP reaches the last element, the loop ends.
Ex:
1 2 3 4 5 6 7 | <?php $colors = ['red', 'green', 'blue']; foreach ($colors as $color) { echo $color . '<br>'; } |
Output:
1 2 3 | red green blue |
With an associative array
Syntax:
foreach ($array_name as $key => $value) {
//process element here;
}
When PHP encounters the foreach
statement, it accesses the first element and assigns:
- The key of the element to the
$key
variable. - The value of the element to the
$value
variable.
In each iteration, PHP assigns the key and value of the next element to the variables ($key
and $value
) that follows the as
keyword. If the last element is reached, PHP ends the loop.
Ex:
1 2 3 4 5 6 7 8 9 10 11 12 13 | <?php $capitals = [ 'Japan' => 'Tokyo', 'France' => 'Paris', 'Germany' => 'Berlin', 'United Kingdom' => 'London', 'United States' => 'Washington D.C.' ]; foreach ($capitals as $country => $capital) { echo "The capital city of {$country} is $capital" . '<br>'; } |
Output:
1 2 3 4 5 | The capital city of Japan is Tokyo The capital city of France is Paris The capital city of Germany is Berlin The capital city of United Kingdom is London The capital city of United States is Washington D.C. |