If statement
Introduction
The if statement allows you to execute a statement if an expression evaluates to true.
if ( expression )
statement;
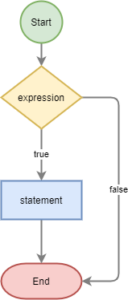
Ex:
1 2 3 4 5 | <?php $is_admin = true; if ($is_admin) echo 'Welcome, admin!'; |
Since $is_admin
is true
, the script outputs the following message:
1 | Welcome, admin! |
Nesting if statements
<?php
if ( expression1 ) {
// do something
if ( expression2 ) {
// do other things
}
}
Ex:
1 2 3 4 5 6 7 8 9 10 11 | <?php $is_admin = true; $can_approve = true; if ($is_admin) { echo 'Welcome, admin!'; if ($can_approve) { echo 'Please approve the pending items'; } } |
Embed if statement in HTML
To embed an if
statement in an HTML document, you can use the above syntax. However, PHP provides a better syntax that allows you to mix the if statement with HTML nicely:
<!– HTML code here –>
<?php endif; ?>
Ex:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>PHP if Statement Demo</title> </head> <body> <?php $is_admin = true; ?> <?php if ( $is_admin ) : ?> <a href="#">Edit</a> <?php endif; ?> <a href="#">View</a> </body> </html> |
A common mistake with the PHP if statement
A common mistake that you may have is to use the wrong operator in the if
statement.
Ex:
1 2 3 4 5 6 | <?php $checked = 'on'; if( $checked = 'off' ) { echo 'The checkbox has not been checked'; } |
This script shows a message if the $checke
d is 'off'
. However, the expression in the if
statement is an assignment, not a comparison:
1 | $checked = 'off' |
This expression assigns the literal string 'off'
to the $checked
variable and returns that variable. It doesn’t compare the value of the $checked
variable with the 'off'
value. Therefore, the expression always evaluates to true
, which is not correct.
To avoid this error, you can place the value first before the comparison operator and the variable after the comparison operator like this:
1 2 3 4 5 6 | <?php $checked = 'on'; if('off' == $checked ) { echo 'The checkbox has not been checked'; } |
If you accidentally use the assignment operator (=), PHP will raise a syntax error instead:
1 2 3 4 5 6 | <?php $checked = 'on'; if ('off' = $checked) { echo 'The checkbox has not been checked'; } //Parse error: syntax error, unexpected '=' ... |
If … else statement
Introduction
if ( expression ) {
// code block
} else {
// another code block
}
In this syntax, if the expression
is true
, PHP executes the code block that follows the if
clause. If the expression
is false
, PHP executes the code block that follows the else
keyword.
Ex:
1 2 3 4 5 6 7 8 9 | <?php $is_authenticated = false; if ( $is_authenticated ) { echo 'Welcome!'; } else { echo 'You are not authorized to access this page.' } //You are not authorized to access <span class="hljs-keyword">this</span> page. |
Embed if…else statement in HTML
Like the if
statement, you can mix the if...else
statement with HTML nicely using the alternative syntax:
<!–Show HTML code when expression is true –>
<?php else: ?>
<!–Show HTML code when expression is false –>
<?php endif ?>
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>PHP if Statement Demo</title> </head> <body> <?php $is_authenticated = true; ?> <?php if ($is_authenticated) : ?> <a href="#">Logout</a> <?php else: ?> <a href="#">Login</a> <?php endif ?> </body> </html> |
If…elseif statement
Introduction
PHP evaluates the expression1
and execute the code block in the if
clause if the expression1
is true
.
If the expression1
is false
, the PHP
evaluates the expression2
in the next elseif
clause. If the result is true
, then PHP executes the statement in that elseif
block. Otherwise, PHP evaluates the expression3
.
If the expression3
is true
, PHP executes the block that follows the elseif
clause. Otherwise, PHP ignores it.
Note:
When anif
statement has multipleelseif
clauses, theelseif
will execute only if the expression in the precedingif
orelseif
clause evaluates tofalse
.
Ex:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | <?php $x = 10; $y = 20; if ($x > $y) { $message = 'x is greater than y'; } elseif ($x < $y) { $message = 'x is less than y'; } else { $message = 'x is equal to y'; } echo $message; |
Output:
1 | x is less than y |
if elseif alternative syntax
if (expression):
statement;
elseif (expression2):
statement;
elseif (expression3):
statement;
endif;
- Use a semicolon (:) after each condition following the
if
orelseif
keyword. - Use the
endif
keyword instead of a curly brace (}
) at the end of theif
statement.
Ex:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 | <?php $x = 10; $y = 20; if ($x > $y) : $message = 'x is greater than y'; elseif ($x < $y): $message = 'x is less than y'; else: $message = 'x is equal to y'; endif; echo $message; |