There may be a situation, when you need to execute a block of code several number of times. In general, statements are executed sequentially: The first statement in a function is executed first, followed by the second, and so on.
A loop statement allows us to execute a statement or group of statements multiple times.
Type | Description |
---|---|
while | Repeats a statement or group of statements while a given condition is true. It tests the condition before executing the loop body. |
for | Execute a sequence of statements multiple times and abbreviates the code that manages the loop variable. |
do…while | Like a ‘while’ statement, except that it tests the condition at the end of the loop body. |
nested loop | You can use one or more loop inside any another ‘while’, ‘for’ or ‘do..while’ loop. |
Loop Control Statements
Loop control statements change execution from its normal sequence. When execution leaves a scope, all automatic objects that were created in that scope are destroyed.
C++ supports the following control statements.
Statement | Description |
---|---|
Break | Terminates the loop or switch statement and transfers execution to the statement immediately following the loop or switch. |
Continue | Causes the loop to skip the remainder of its body and immediately retest its condition prior to reiterating. |
Goto | Transfers control to the labeled statement. Though it is not advised to use goto statement in your program. |
While Loop
Syntax:
while (test_expression)
{
// statements
update_expression;
}
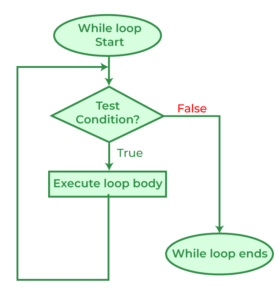
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | #include <iostream> using namespace std; int main() { // initialization expression int i = 1; // test expression while (i < 6) { cout << "Hello World\n"; // update expression i++; } return 0; } |
Output:
1 2 3 4 5 | Hello World Hello World Hello World Hello World Hello World |
For Loop
Syntax:
// body of-loop
}
- initialization – initializes variables and is executed only once.
- condition – if true, the body of for loop is executed if false, the for loop is terminated.
- update – updates the value of initialized variables and again checks the condition.
Ex:
1 2 3 4 5 6 7 8 9 | #include <iostream> using namespace std; int main() { for(int i=5;i>=0;i--) cout<<i<<" "; return 0; } |
Output:
1 | 5 4 3 2 1 0 |
Flow Diagram of for loop:
Do … while Loop
In Do-while loops also the loop execution is terminated on the basis of test conditions. The main difference between a do-while loop and the while loop is in the do-while loop the condition is tested at the end of the loop body, i.e do-while loop is exit controlled whereas the other two loops are entry-controlled loops.
Note:
In a do-while loop, the loop body will execute at least once irrespective of the test condition.
Syntax:
do
{
// statements
update_expression;
}
while (test_expression);
Note:
Notice the semi – colon( ; )in the end of loop.
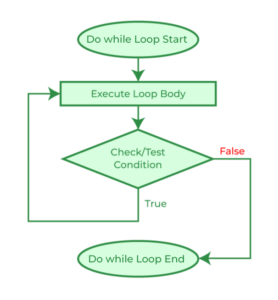
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | #include <iostream> using namespace std; int main() { int i = 2; // Initialization expression do { // loop body cout << "Hello World\n"; // update expression i++; } while (i < 1); // test expression return 0; } |
Output:
1 | Hello World |